Todayโs Outline
- Loop
- Programming styles for control flow
Loop
while
int x, max;
max = 0;
cout << "Enter a positive integer. ";
cout << "Type 0 to quit.\\n";
cin >> x;
while (x != 0) {
if (x > max) {
max = x;
}
cout << "Enter a positive integer. ";
cout << "Type 0 to quit.\\n";
cin >> x;
}
do-while
int x, max;
max = 0;
do {
cout << "Enter a positive integer. ";
cout << "Type 0 to quit.\\n";
cin >> x;
if (x > max) {
max = x;
}
} while (x != 0);
- do-while is better suited for loops that require at least one iteration
for
int i; // Declare iteration variable outside
for (i = 0; i < 10; i++) {
if (i % 2 == 0) {
cout << i << endl;
}
}
goto statement
int main() {
int num;
cin >> num;
if (num % 2 == 0)
goto even;
else
goto odd;
even:
cout << num << " is even\\n";
return 0;
odd:
cout << num << " is odd\\n";
return 0;
}
- bad programming practice
- can be replaced with
break
and continue
Short-Circuit Evaluation with goto
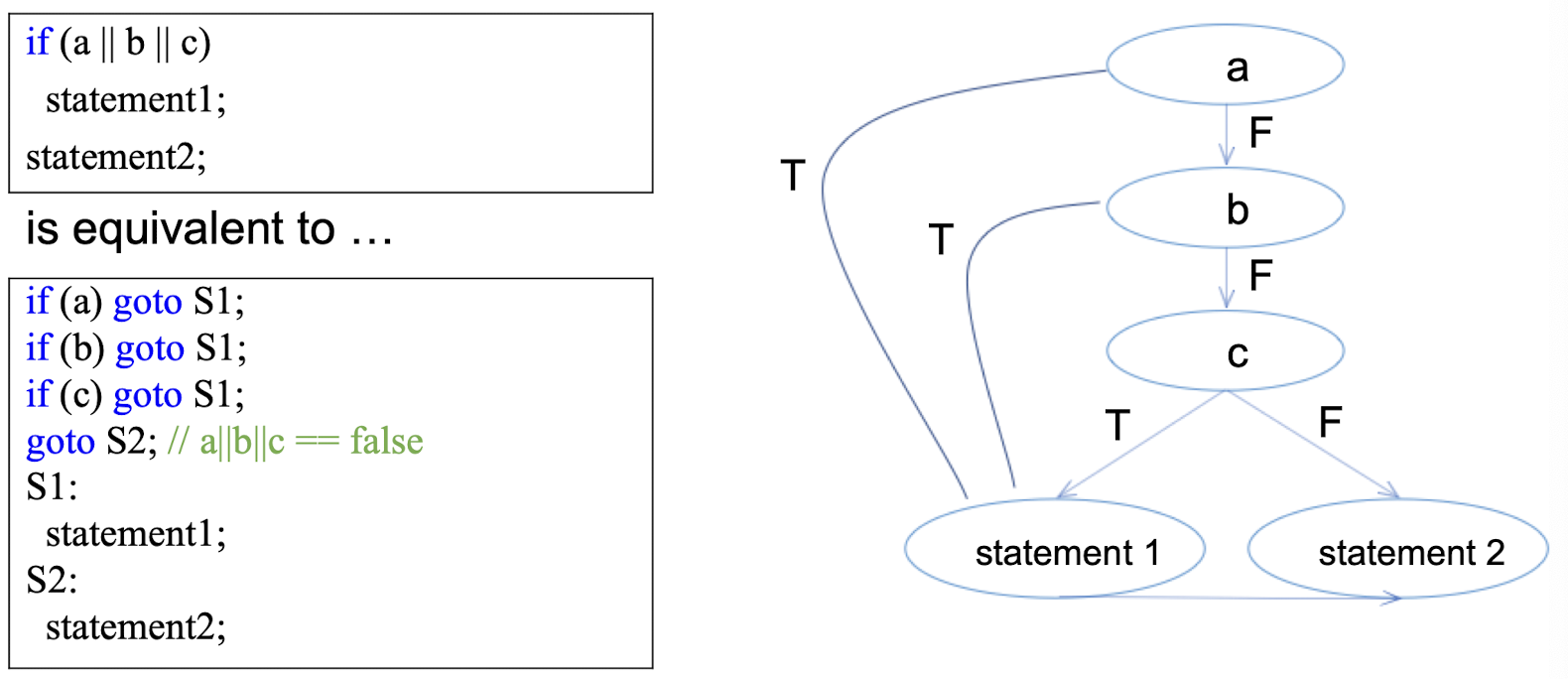
Programming styles for control flow
- Indentation
- if Condition Style