Todayโs Outline
- Recap: char
- C string basics
- Reading and printing C strings
- Common string functions
- Safety of string functions
Recap: char
cstring vs std::string
cstring
- inherited from the C lang
string
- class defined in std lib
#include <string>
- Class and object
C String Basics
C String: '\\0'
- A C string is a char array terminated by
'\\0'
- Used to distinguish a C String from an ordinary char array
'\\0'
== null
char str[20] = "Hello World"; // '\\0' will be added at the last index
C String: Declaration and Initialization
// With initialization
char studentID[] = "51234567";
char HKID[] = "a123456(7)";
// Without initialization
char studentID[8+1];
char HKID[10+1];
// Cannot initialize after the declaration
char name[10];
name = "john"; // error
C String: Storage
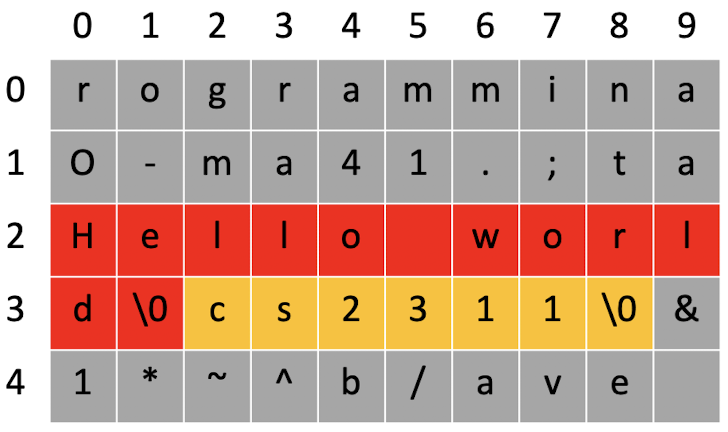
char s1[] = "Hello World"; // s1 = 20
char s2[] = "cs2311"; // s2 = 32
Passing String to Functions
int count(char s[100], char c) {
int frequency=0;
int i=0;
while (s[i] != '\\0') {
if (s[i] == c) {
frequency++;
}
i++;
}
return frequency;
}
int main() {
char T[100] = โhello worldโ;
char t = โaโ;
cout << count(T, t) << endl;
return 0;
}
Reading and Printing C strings
int main() {
char word[5];
// we can use 'cin' to initialize the string after declaration
cin >> word;
cout << word;
return 0;
}
Printing C Strings
int main() {
char s1[] = "abc";
char s2[] = "def";
s1[3] = '+';
cout << s1 << endl << s2 << endl;
return 0;
// abc+def
// def
}
Reading C Strings
char s1[20], s2[20];
// 'cin' will terminate when whitespace is encountered
// whitespace = space, teb, newline, ...
cin >> s1; // input = "hello world" / s1 = "hello\\0"
cin >> s2; // buffer = "world" / s2 = "world\\0"
cout << s1; // will print "hello"
cout << s2; // will print "world"
Reading a Line: get() Loop
char s[20];
int i = 0;
char c;
do {
cin.get(c); // input = "hello world" / c = 'h'
str[i++] = c;
} while (c != '\\n' && i < 20);
Reading a Line: getline()
char s[20];
cin.getline(s, 20);
int main() {
char s[5];
int i = 0;
while (true) {
cin.getline(s, 5); // will accept 4 characters + eofbit flag set
cin.clear(); // reset flag
cout << i++ << ": " << s << endl;
}
return 0;
// 0: 1234
// 1: 5
}
Common cstring Functions
strlen()
char s[20] = "Hello World!";
int len = strlen(s);
int siz = sizeof(s);
cout << len << endl; // 12
cout << siz << endl; // 20
strlen(str)
- returns the number of chars
strcpy()
char s1[6];
strcpy(s1, "hello");
char s2[6];
strcpy(s2, s1);
s2[0] = 'c';
cout << s1 << endl; // hello
cout << s2 << endl; // cello
strcpy(dst, src)
- copies src
into dst
strcat()
char s[13];
strcpy(s, "hello ");
strcat(s, "world!");
cout << s; // "hello world!
strcat(dst, src)
- concatenates src
into dst
strcmp()
cout << strcmp("abc", "abc") << endl; // 0
cout << strcmp("abc", "abcd") << endl; // -1
cout << strcmp("abcd", "abc") << endl; // 1
cout << strcmp("abc", "abd") << endl; // -1
strcmp(s1, s2)
- compare s1
and s2
until encountering different characters
< 0
if s1 < s2
in alphabet
> 0
if s1 > s2
in alphabet
0
if s1 == s2
Other String Functions
strncpy(dst, src, n)
strncat(dst, src, n)
strncmp(str1, str2, n)
strchr(str, ch)
/ strrchr(str, ch)
strstr(haystack, needle)
strspn(str, accept)
strcspn(str, reject)
Safety of String Functions
char s1[14];
strcpy(s1, "hello, world!");
char s2[6];
strcpy(s2, s1); // s2 = "hello," / other program memory = " world!\\0"
Additional Notes
strcpy
and strcat
are considered unsafe
, as they donโt check memory boundary
- Use
strcpy_s
and strcat_s
instead